Observability for Cohere with Langfuse
This guide shows you how to integrate Cohere with Langfuse using the OpenAI SDK Compatibility API. Trace and monitor your applications seamlessly.
What is Cohere? Cohere is an AI platform that provides state-of-the-art language models via API, allowing developers to build applications with natural language understanding capabilities.
What is Langfuse? Langfuse is an open source LLM engineering platform for tracing, monitoring, and debugging LLM applications.
Step 1: Install Dependencies
Ensure you have the necessary Python packages installed:
%pip install openai langfuse
Step 2: Set Up Environment Variables
import os
# Get keys for your project from the project settings page
# https://cloud.langfuse.com
os.environ["LANGFUSE_PUBLIC_KEY"] = "pk-lf..."
os.environ["LANGFUSE_SECRET_KEY"] = "sk-lf..."
os.environ["LANGFUSE_HOST"] = "https://cloud.langfuse.com" # 🇪🇺 EU region
# os.environ["LANGFUSE_HOST"] = "https://us.cloud.langfuse.com" # 🇺🇸 US region
# Set your Cohere API key from your Cohere account settings
os.environ["COHERE_API_KEY"] = "..."
Step 3: Use Cohere with the OpenAI SDK
Leverage the Compatibility API by replacing the base URL with Cohere’s endpoint when initializing the client.
# Instead of importing openai directly, use Langfuse's drop-in replacement
from langfuse.openai import openai
client = openai.OpenAI(
api_key=os.environ.get("COHERE_API_KEY"),
base_url="https://api.cohere.ai/compatibility/v1" # Cohere Compatibility API endpoint
)
Step 4: Run an Example
The example below demonstrates a basic chat completion request. All API calls are automatically traced by Langfuse.
response = client.chat.completions.create(
model="command-r7b-12-2024", # Replace with the desired Cohere model
messages=[
{"role": "system", "content": "You are an assistant."},
{"role": "user", "content": "Tell me about the benefits of using Cohere with Langfuse."}
],
name="Cohere-Trace"
)
print(response.choices[0].message.content)
Using Cohere's Command model alongside Langfuse, a powerful observability tool for LLMs, can bring several advantages to your workflow:
- **Enhanced Data Analysis:** Langfuse provides a comprehensive set of features for tracking and analyzing LLM outputs. By integrating Cohere's Command model with Langfuse, you can gain insights into the model's performance, including response quality, latency, and cost. This analysis helps in optimizing the model's usage and identifying areas for improvement.
- **Customizable Feedback Loops:** Langfuse allows you to create customizable feedback loops, which are essential for training and refining language models. You can design specific feedback mechanisms to evaluate and improve the outputs generated by Cohere's Command model. This ensures that the model learns from user feedback and adapts to meet specific requirements.
- **Real-time Monitoring:** With Langfuse, you can set up real-time monitoring to track the performance of Cohere's Command model as it processes requests. This feature enables you to quickly identify and address any issues or anomalies, ensuring a consistent and reliable user experience.
- **Advanced Error Handling:** Langfuse offers advanced error-handling capabilities, allowing you to define custom error responses and notifications. This is particularly useful when integrating Cohere's model into production environments, as it helps in managing potential errors and ensuring graceful degradation of the system.
- **User Experience Improvement:** By utilizing Langfuse's analytics and feedback collection, you can gather valuable user feedback on the Cohere model's responses. This feedback can be used to enhance the model's capabilities, improve response accuracy, and tailor the output to better meet user expectations.
- **Scalability and Cost Management:** Langfuse provides tools to monitor and manage the cost of using Cohere's Command model, especially in large-scale applications. You can track usage, set usage-based thresholds, and optimize costs, ensuring efficient resource utilization.
- **Integration and Customization:** Langfuse offers a flexible integration process, allowing you to seamlessly connect Cohere's Command model with your existing systems and workflows. This customization ensures that the solution fits your specific needs and integrates well with your current infrastructure.
By combining Cohere's Command model with Langfuse's observability and feedback capabilities, you can create a robust and efficient LLM-powered system, ensuring improved performance, user satisfaction, and cost-effectiveness.
Step 5: Enhance Tracing (Optional)
You can enhance your Cohere traces:
- Add metadata, tags, log levels and user IDs to traces
- Group traces by sessions
@observe()
decorator to trace additional application logic- Use Langfuse Prompt Management and link prompts to traces
- Add score to traces
Visit the OpenAI SDK cookbook to see more examples on passing additional parameters. Find out more about Langfuse Evaluations and Prompt Management in the Langfuse documentation.
Step 6: See Traces in Langfuse
After running the example, log in to Langfuse to view the detailed traces, including request parameters, response content, token usage, and latency metrics.
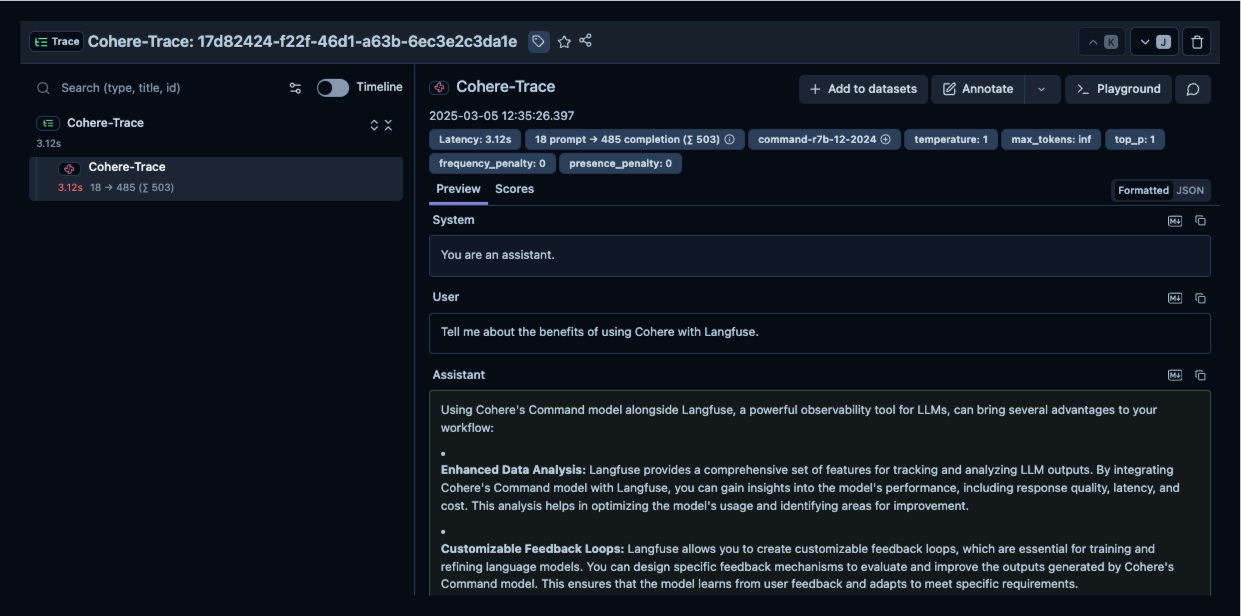